What is the difference between %w and %W array literals in Ruby
This minipost will explain in detail the difference between the lower case %w
and the uppercase %W
percent string literals in Ruby on string interpolation cases and escape sequences.
By definition the lower case %w
string literal creates an Array
of String
-s if you supply words separated by whitespaces inside the brackets.
The following declaration:
%w[a b c d]
will output:
["a", "b", "c", "d']
However, the %w
literal treats the supplied strings as single-quoted and as a result, it constructs an Array
of strings that have not been escaped or interpolated.
To demonstrate most of the cases and the main difference between %w
and %W
Ruby Array percent literals we will assume the following for the irb session:
a = 'a'
We will execute a %w
construction that contains interpolation, escape sequences and the special case of adding whitespace between two strings.
The following declaration:
%w[a#{a} b#{'b'} c\ d \s \']
will output:
=> ["a\#{a}", "b\#{'b'}", "c d", "\\s", "\\'"]
From the above we can notice the following:
- that we tried to interpolate a String with a variable and a String with another String, however, all the interpolation declaration was not escaped and it evaluated in a string with value
"a\#{a}"
and in a string with"b\#{'b'}"
as the first two items in the Array. - the lower case
%w
literal only adds whitespaces between the supplied strings if and only if you have specified\
(backward slash & whitespace) and not the space literal\s
On the other hand, the upper-case %W
literal treat the supplied strings in the brackets as double-quoted String
-s and thus it will construct an Array
from interpolated string expressions and it will escape sequences prior to the Array creation.
To inspect the behaviour of the the %W
literal we will use the previous example:
%W[a#{a} b#{'b'} c\ d \s \']
and inspect the output:
=> ["aa", "bb", "c d", " ", "'"]
From the above snippet, we can see that %W
percent literal allows string interpolation between a String and a dynamic variable, it escapes sequences and the \
(backward slash & space) sequence that the lower case uses for spaces between Strings.
The lower-case %w
percent literal:
- treats all supplied words in the brackets as single-quoted Strings
- does not interpolate Strings
- does not escape sequences
- escapes only whitespaces by
\
The upper-case %W
percent literal:
- treats all supplied words in the brackets as double-quoted Strings
- allows String interpolation
- escapes sequences
- escapes also whitespaces by
\
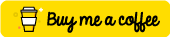