Avoid the use of unless conditional with an else clause in Ruby
In Ruby, there are three ways of negating conditions. The first one is the not
keyword. The second one is with the negating !
(the bang operator of negation). The third and more natural sounding way of negating is the unless
keyword. The keyword unless
, express the exact same semantics as if not (expression)
and if !(expression)
.
So we can summarise the three negating conditions in the following gist:
unless (x == 0)
the same as if not (x == 0)
the same as if !(x == 0)
By using unless
in our code we aim to provide better readability and a more natural sounding in the English of the code. Think about it, unless
sounds clearer than if not
and it's much more easy to understand the logic in the part of code that is involved. Furthermore, Ruby language, allows the use of unless
with an else
clause like in the following gist:
unless (x == 0) puts "Not a Zero" else puts "A Zero" end
The above is a valid example of code that will be parsed without errors or warnings. However, is hard to follow the logic even in the above simple example. In general, if/else
reads much better than unless/else
. The use of unless
with an else
clause is considered a bad practice and should be avoided. By flipping around the logic of the conditional statement above and by replacing the former with the latter statement, we result in a statement with the exact same conditional meaning.
if (x == 0) puts "A Zero" else puts "Not a Zero" end
Note: We also avoided to use the other negating conditionals and just flip the statement to an if/else that is easier to understand by every member of the team. To summarize unless
sounds better and it is used widely as a single conditional modifier. If there is the need of an else
clause, you should refactor to an if/else
statement.
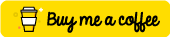